2023. 3. 10. 17:39ㆍ공부 중/Node.js
Node.js - MySQL - 생활코딩
수업소개 이 수업은 Node.js와 MySQL을 이용해서 웹애플리케이션을 만드는 방법에 대한 수업입니다. 수업대상 예를들어 1억 개의 페이지로 이루어진 웹사이트에서 필요한 정보가 파일에 하나하나
opentutorials.org
생활코딩 Node.js - MySQL 강의를 듣고서 작성한 글입니다. 그냥 그렇다고요.
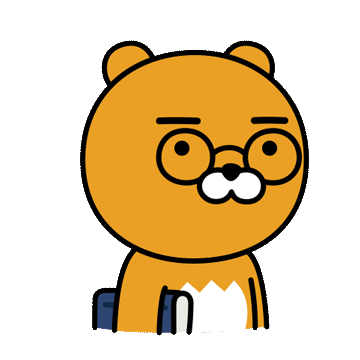
1. file의 한계
소규모의 단순한 기능을 구현하기에는 파일이 좋다.
단순하고 쉽고 특별한 설치나 학습이 필요하지 않다.
하지만 파일은 한계가 있다.
우리가 만든 웹 페이지가 저장한 파일의 수가 커질수록 원하는 파일, 원하는 내용을 찾는 것이 오래 걸린다.
뿐만 아니라 파일은 제목과 본문이라는 두 가지 요소만을 표현할 수 있다.
부가적으로 작성자의 이름, 작성 시간, 공개 상태 등 관련된 정보를 추가하기 어렵다.
그 외에도 보안, 안정성, 성능, 동시성 등 개발자가 직접 관리하기 어려운 문제가 발생하기 쉽다.
2. Database 활용하기
우리는 배울 것도 많고 복잡하지만 DB를 사용할 필요가 있다.
데이터베이스를 사용하면 파일보다 더 많은 정보를 저장하고 관리할 수 있다.
파일 시스템의 한계를 극복해 데이터를 효과적으로 구성, 저장, 활용할 수 있다.
또한 다른 프로그램과 데이터를 공유할 수 있도록 하는 동시성 및 보안 기능을 제공하며, 데이터 검색 및 조작이 쉽고 빠르며 유연하다.
생활코딩 node.js 수업에서는 MySQL을 사용한다.
우리는 Node.js에서 MySQL을 제어하기 위해서 만든 라이브러리에 대해서 학습할 것이다.
3. 실습 준비
실습준비 - 생활코딩
수업소개 실습에 참여하는 방법을 소개합니다. 수업내용 실습코드 이 수업은 WEB2 - Node.js과 DATABASE2 - MySQL을 기반으로 하고 있습니다. 이를 위한 실습코드가 필요합니다. 아래 주소에 준비해 두
opentutorials.org
실습을 위해서 MySQL을 설치하고 database를 세팅했다.
4. node.js에서 mysql 사용하기
node.js 자체에서 제공하는 모듈 중에는 mysql을 제어하기 위한 모듈은 없다.
npm에서 추가적인 모듈을 설치해줘야 한다.
mysql
A node.js driver for mysql. It is written in JavaScript, does not require compiling, and is 100% MIT licensed.. Latest version: 2.18.1, last published: 3 years ago. Start using mysql in your project by running `npm i mysql`. There are 7032 other projects i
www.npmjs.com
Node.js MySQL
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
npm install mysql
npm install mysql2 #mysql의 버전이 8 이상인 경우 추천
설치를 완료한다.
아래는 예시다.
const mysql = require('mysql'); //= require('mysql2');
const connection = mysql.createConnection({
host : 'localhost',
user : 'me',
password : 'secret',
database : 'my_db'
});
connection.connect();
connection.query('SELECT 1 + 1 AS solution', function (error, results, fields) {
if (error) throw error;
console.log('The solution is: ', results[0].solution);
});
connection.end();
가. Create Connection
const mysql = require('mysql');
const connection = mysql.createConnection({
host : 'localhost',
user : 'me',
password : 'secret',
database : 'my_db'
});
connection.connect();
...
connection.end();
const mysql = require('mysql');
: 설치한 mysql 모듈을 import한다.const connection = mysql.createConnection({ … });
: mysql에 연결하고 이를 객체로 만든다.host : 'localhost',
: 연결하려는 데이터베이스의 호스트 이름입니다. (기본값:localhost
)user : 'me',
: 인증할 MySQL 사용자입니다.password : 'secret',
: 해당 MySQL 사용자의 비밀번호입니다.database : 'my_db'
: 이 연결에 사용할 데이터베이스의 이름입니다 (선택 사항).
나. Query a Database
...
connection.connect();
connection.query('SELECT 1 + 1 AS solution', function (error, results, fields) {
if (error) throw error;
console.log('The solution is: ', results[0].solution);
});
connection.end();
...
connection.query('SQL 쿼리', function (error, results, fields) { … });
: 실행하고자 하는 쿼리문을 문자열로 입력한다. 그리고 결과를 받아서 사용할 콜백함수를 작성한다.error
: Error if one occurred during the query.results
: The results of the query. 객체의 배열이다.result[숫자].필드값
fieds
: information about the returned results fields.
var queryData = url.parse(_url, true).query;
...
connection.query(`SELECT * FROM topic WHERE id = ${queryData.id}`, function (error, results, fields) { ... });
topic
이라는 테이블에서 id
가 queryData.id
와 같은 튜플의 모든 속성을 가져오는 SELECT
문이다.
보안을 위해서 아래와 같이 사용할 수 있다.
connection.query(`SELECT * FROM topic WHERE id = ?`, [queryData.id], function (error, results, fields) { ... });
입력값을 ?
로 남겨놓고 두 번째 인자에 배열로 전달하면 SQL injection와 같이 불순한 의도를 가진 입력값에 대하여 자동으로 처리해 준다.
다. Streaming query rows
만약 많은 양의 rows를 select하고 이를 오는 족족 처리하고 싶다면 다음과 같이 코드를 작성하면 된다.
var query = connection.query('SELECT * FROM posts');
query
.on('error', function(err) {
// Handle error, an 'end' event will be emitted after this as well
})
.on('fields', function(fields) {
// the field packets for the rows to follow
})
.on('result', function(row) {
// Pausing the connnection is useful if your processing involves I/O
connection.pause();
processRow(row, function() {
connection.resume();
});
})
.on('end', function() {
// all rows have been received
});
더 궁금한 점은 아래에서 찾아볼 것.
mysql
A node.js driver for mysql. It is written in JavaScript, does not require compiling, and is 100% MIT licensed.. Latest version: 2.18.1, last published: 3 years ago. Start using mysql in your project by running `npm i mysql`. There are 7032 other projects i
www.npmjs.com
mysql2
fast mysql driver. Implements core protocol, prepared statements, ssl and compression in native JS. Latest version: 3.2.0, last published: 10 days ago. Start using mysql2 in your project by running `npm i mysql2`. There are 3493 other projects in the npm r
www.npmjs.com
'공부 중 > Node.js' 카테고리의 다른 글
[Node.js] MySQL로 기능 구현 (Create, Read) (0) | 2023.03.27 |
---|---|
[Node.js] npm install mysql2 (0) | 2023.03.13 |
[Nodejs] TypeError: Cannot convert undefined or null to object (0) | 2023.03.07 |
[Jest] Setup and Teardown (0) | 2023.03.01 |
[Jest] Testing Asynchronous Code (0) | 2023.03.01 |