2022-08-18 JS_5 (배열, 반복문, 콘솔, 함수)
2022. 8. 19. 00:46ㆍFE/JavaScript
배열과 반복문의 활용 - 생활코딩
소스코드 변경사항
opentutorials.org
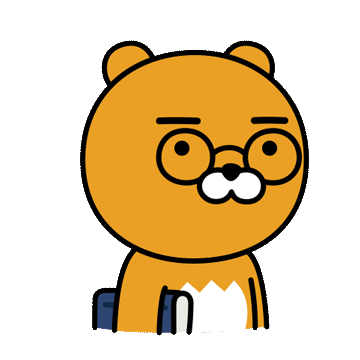
이 글은 이고잉님의 오픈튜토리얼 강의를 듣고 정리한 내용입니다.
1. 배열과 반복문
정통적으로 배열과 반복문은 같이 사용되는 경우가 많다.
가. 실습 : 리스트 작성
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Loop & Array</h1>
<h2>count</h2>
<ul>
<li>one</li>
<li>two</li>
<li>three</li>
<li>four</li>
</ul>
</body>
</html>
같은 효과를 내는 더 효율적인 방법이 있다.
...
<ul>
<li>one</li>
<li>two</li>
<li>three</li>
<li>four</li>
</ul>
...
해당 코드를 배열과 반복문을 이용해서 대체하면 유지보수 측면, 중복 최소화, 가족성 측면에서 유리하다.
...
<ul>
<script>
var array = ['one', 'two', 'three', 'four'];
var i = 0;
while(i < array.length){
document.write('<li>'+ array[i]+'</li>');
i = i + 1;
}
</script>
</ul>
...
배열의 크기만큼 배열의 값을 리스트로 작성한다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Loop & Array</h1>
<h2>count</h2>
<ul>
<script>
var array = ['one', 'two', 'three', 'four'];
var i = 0;
while(i < array.length){
document.write('<li>'+ array[i]+'</li>');
i = i + 1;
}
</script>
</ul>
</body>
</html>
2. 콘솔
개발자 도구를 잘 다루면 편하다.
우클릭 → ‘검사’ 클릭 → ‘console’
콘솔창을 통해 자바스크립트 코드를 즉석에서 작성, 실행할 수 있다.
alert('Hi');
상단에 경고창을 생성한다.
경고 메시지의 내용은 괄호 안에 문자형으로 입력하면 된다.
console.log('Hi');
콘솔창에 출력한다.
shift+enter : 줄 바꿈.
![]() |
![]() |
줄을 바꿈으로써 보다 긴 코드를 작성할 수 있다.
3. 함수
가. 기본 문법
...
<script>
function sum(left, right){
document.write(left + right + '<br>');
}
sum(1, 1);
sum(2, 3);
</script>
...
나. Return하는 방법
...
<script>
function sum2(left ,right) {
return left + right;
}
sum2(1, 1);
sum2(2, 3);
</script>
다. void? undefined?
2024년 1월 13일 추가
Javascript의 함수는 무조건 반환값을 가진다.
그래서 void가 없다.
특별한 반환값이 할당되어있지 않았다면 undefined를 반환한다.
이는 "값이 아직 할당되어있지않음"을 뜻한다.
라. 유용한 함수들
1. setTimeout, clearTimeout
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
var loop;
const f1 = function(){
loop = window.setTimeout("alert('확인')", 3000);
}
const f2 = function(){
console.log("f2");
clearTimeout(loop);
}
console.log("res : ", f2());
</script>
</head>
<body>
<button onclick="f1()">시작</button>
<button onclick="f2()">정지</button>
</body>
</html>
마. Event
이벤트를 처리하는 두가지 방식.
<!-- on???? 사용 -->
<script>
var loop;
const f1 = function(){
loop = window.setTimeout("alert('확인')", 3000);
}
const f2 = function(){
console.log("f2");
clearTimeout(loop);
}
console.log("res : ", f2());
</script>
...
<button onclick="f1()">시작</button>
<--! 이벤트 리스너 사용 -->
<script>
var loop;
const f1 = function(){
loop = window.setTimeout("alert('확인')", 3000);
}
const f2 = function(){
console.log("f2");
clearTimeout(loop);
}
console.log("res : ", f2());
</script>
...
<button id="s">시작</button>
<script>
document.querySelector("#s").addEventListener("click", f1);
</script>
'FE > JavaScript' 카테고리의 다른 글
[JavaScript] var과 let (0) | 2023.02.10 |
---|---|
2022-08-22 JS_6 (0) | 2022.08.22 |
2022-08-16 JS_4 (0) | 2022.08.17 |
2022-08-14 JS_3 (0) | 2022.08.16 |
2022-08-14 JS_2 (0) | 2022.08.14 |